Simulations¶
In this tutorial, we explore
The mathematical representations of the simulations
Plots showing each simulation
Mathematical Equations¶
Simulations for the power curves were generated using the following equations:
Linear\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\):
Exponential\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\):
Cubic\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\):
Joint Normal\(\left ( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}^p\): Let \(\rho = 1/2 p\), \(I_p\) be the identity matrix of size \(p \times p\), \(J_p\) be the matrix of ones of size \(p \times p\), and \(\Sigma = \begin{bmatrix} I_p & \rho J_p \\ \rho J_p & \left(1 + 0.5 \kappa \right) I_p\\ \end{bmatrix}\). Then,
Step Function\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\):
where \(\mathcal{I}\) is the indicator function, that is \(\mathcal{I} \left( z \right)\) is unity whenever \(z\) is true, and \(0\) otherwise.
Quadratic\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\):
W-Shape\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\): For \(U \sim {\mathcal{U} \left( -1, 1 \right)}^p\),
Spiral\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\): For \(U \sim \mathcal{U} \left( 0, 5 \right)\), \(\epsilon \sim \mathcal{N} \left( 0, 1 \right)\),
Uncorrelated Bernoulli\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\): For \(U \sim \mathcal{B} \left( 0.5 \right)\), \(\epsilon_1 \sim \mathcal{N} \left( 0, I_p \right)\), \(\epsilon_2 \sim \mathcal{N} \left( 0, 1 \right)\),
Logarithmic\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}^p\): For \(\epsilon \sim \mathcal{N} \left( 0, I_p \right)\),
Fourth Root\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\):
Sine Period 4\(\pi\) \(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}^p\): For \(U \sim \mathcal{U} \left( -1, 1 \right)\), \(V \sim {\mathcal{N} \left( 0, 1 \right)}^p\), \(\theta = 4 \pi\),
Sine Period 16\(\pi\) \(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}^p\): Same as above except \(\theta = 16 \pi\) and the noise on \(Y\) is changed to \(0.5 \kappa \epsilon\).
Square\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}^p\): For \(U \sim \mathcal{U} \left( -1, 1 \right)\), \(V \sim \mathcal{U} \left( -1, 1 \right)\), \(\epsilon \sim {\mathcal{N} \left( 0, 1 \right)}^p\), \(\theta = -\frac{\pi}{8}\),
Diamond\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}^p\): Same as above except \(\theta = \pi/4\).
Two Parabolas\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\): For \(\epsilon \sim \mathcal{U} \left( 0, 1 \right)\), \(U \sim \mathcal{B} \left( 0.5 \right)\),
Circle\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\): For \(U \sim {\mathcal{U} \left( -1, 1 \right)}^p\), \(\epsilon \sim \mathcal{N} \left( 0, I_p \right)\), \(r = 1\),
Ellipse\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}^p\): Same as above except \(r = 5\).
Multiplicative Noise\(\left( x, y \right) \in \mathbb{R}^p \times \mathbb{R}^p\): \(u \sim \mathcal{N} \left( 0, I_p \right)\),
Multimodal Independence\(\left( X, Y \right) \in \mathbb{R}^p \times \mathbb{R}\): For \(U \sim \mathcal{N} \left( 0, I_p \right)\), \(V \sim \mathcal{N} \left( 0, I_p \right)\), \(U' \sim {\mathcal{B} \left( 0.5 \right)}^p\), \(V' \sim {\mathcal{B} \left( 0.5 \right)}^p\),
Plots¶
Let’s import some useful packages and create a function that plots our simulated 1D data, to ensure consistency in these examples, we set the seed:
[1]:
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt; plt.style.use('classic')
import seaborn as sns; sns.set(style="white")
from mgcpy.benchmarks.simulations import *
np.random.seed(12345678)
[4]:
def plot_sims(sim_name, sim_func):
"""
Plots all of the simulations
"""
if sim_name == 'Sine (16$\pi$)':
x, y = sim_func(num_samp=1000, num_dim=1, noise=0, period=16*np.pi)
elif sim_name == 'Ellipse':
x, y = sim_func(num_samp=1000, num_dim=1, noise=0, radius=5)
elif sim_name == 'Diamond':
x, y = sim_func(num_samp=1000, num_dim=1, noise=0, period=-np.pi/4)
elif sim_name == 'Multiplicative Noise' or sim_name == 'Multimodal Independence':
x, y = sim_func(num_samp=1000, num_dim=1)
else:
x, y = sim_func(num_samp=1000, num_dim=1, noise=0)
# Normalize
x = x / np.max(x)
y = y / np.max(y)
fig = plt.figure(figsize=(8,8))
fig.suptitle(sim_name + " Simulation", fontsize=17)
ax = sns.scatterplot(x=x[:,0], y=y[:,0])
ax.set_xlabel('Simulated X', fontsize=15)
ax.set_ylabel('Simulated Y', fontsize=15)
plt.axis('equal')
plt.xticks(fontsize=15)
plt.yticks(fontsize=15)
plt.show()
Simultions are randomly generated with an \(x\) which is \((n \times d)\) and \(y\) which is \((n \times 1)\) that have 2 required parameters: num_samp
or the number of samples, and num_dim
or the number of dimensions. Optional parameters can be set based on the documentation. Visualizations of are shown below with and without the noise. Here are all the simulations:
[5]:
sim_func = [linear_sim, exp_sim, cub_sim, joint_sim, step_sim, quad_sim, w_sim, spiral_sim, ubern_sim, log_sim,
root_sim, sin_sim, sin_sim, square_sim, two_parab_sim, circle_sim, circle_sim, square_sim,
multi_noise_sim, multi_indep_sim]
sim_name = ['Linear', 'Exponential', 'Cubic', 'Joint Normal', 'Step', 'Quadratic', 'W-Shaped', 'Spiral',
'Uncorrelated Bernoulli', 'Logarithmic', 'Fourth Root', 'Sine (4$\pi$)', 'Sine (16$\pi$)', 'Square',
'Two Parabolas', 'Circle', 'Ellipse', 'Diamond', 'Multiplicative Noise', 'Multimodal Independence']
for i in range(len(sim_func)):
plot_sims(sim_name[i], sim_func[i])
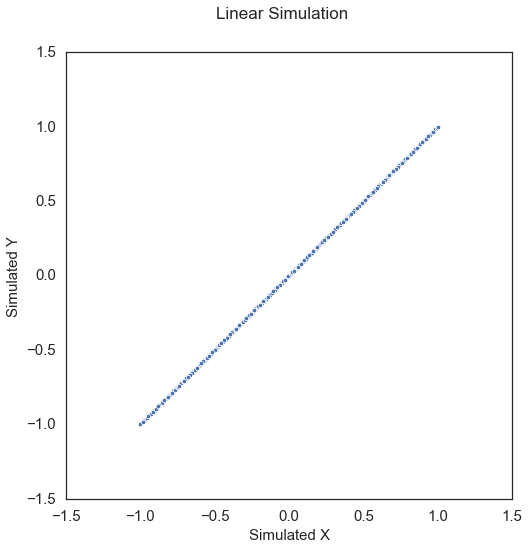
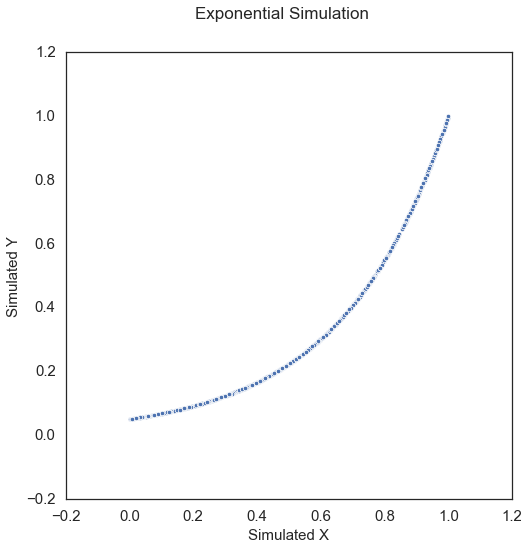
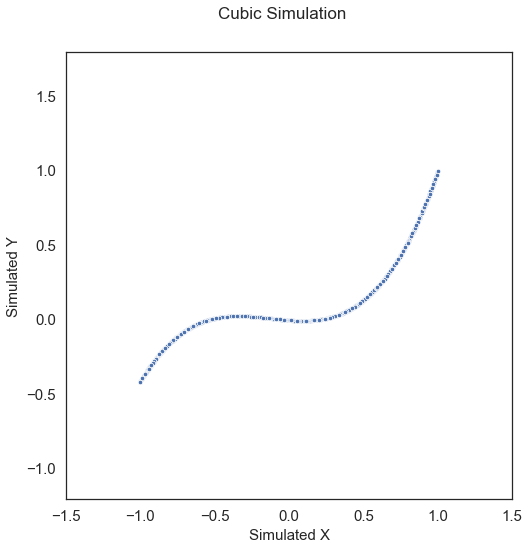
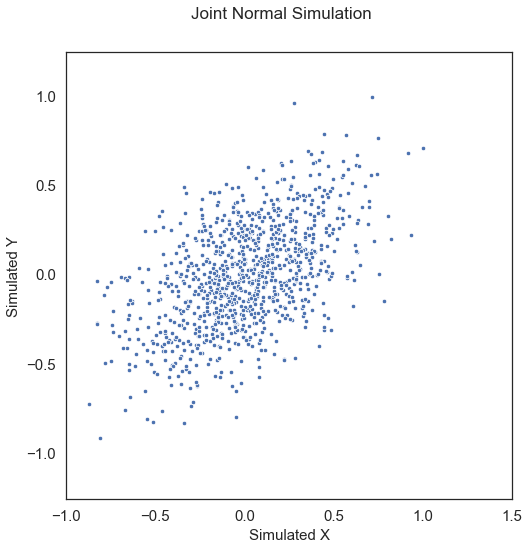
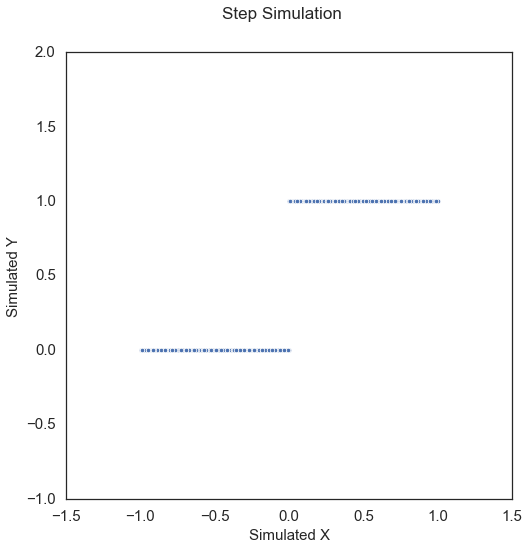
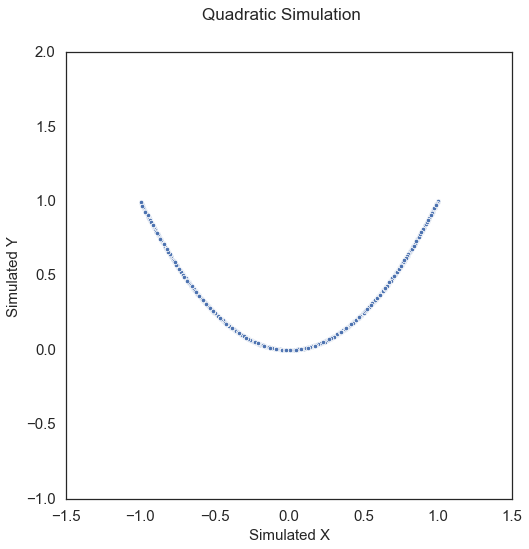
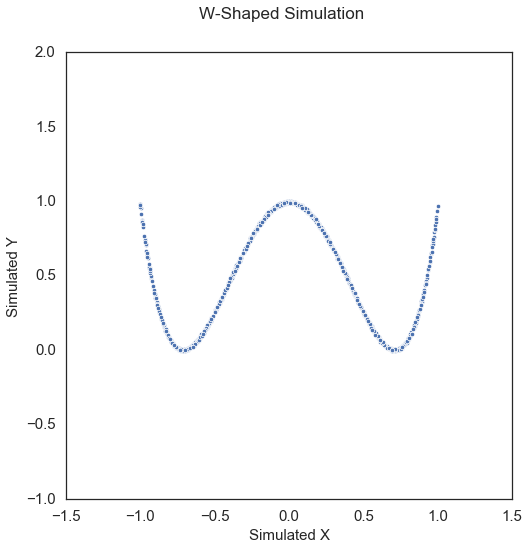
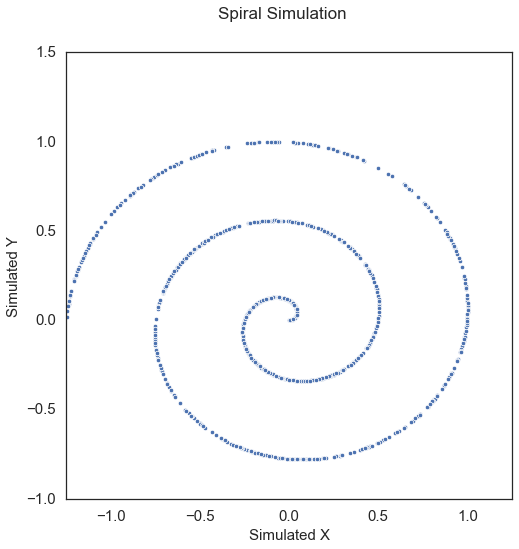
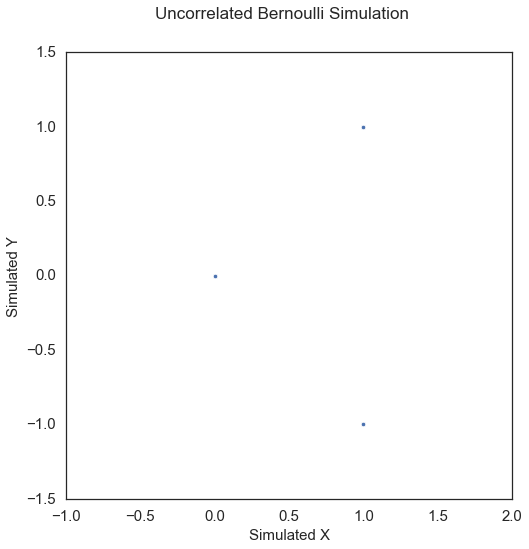
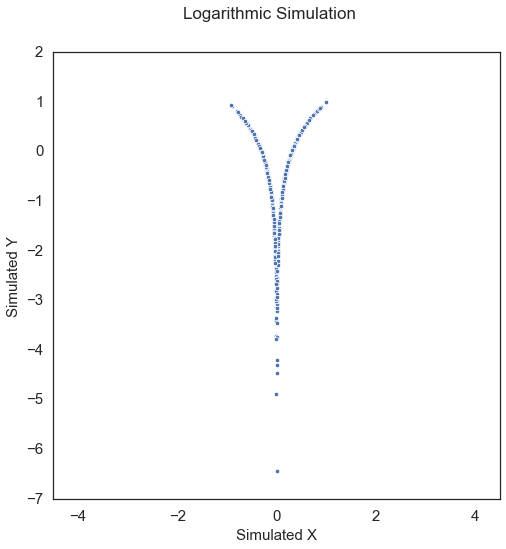
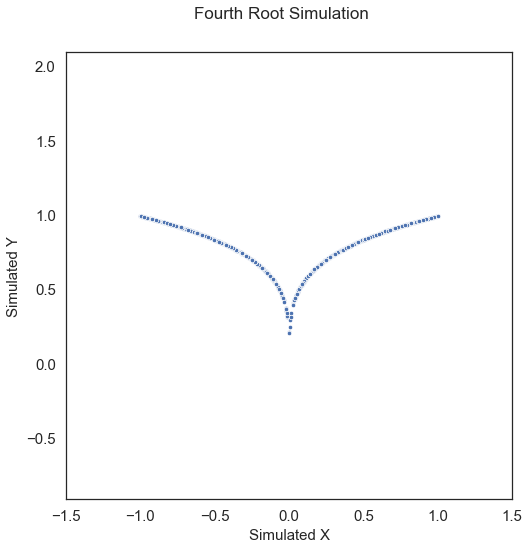
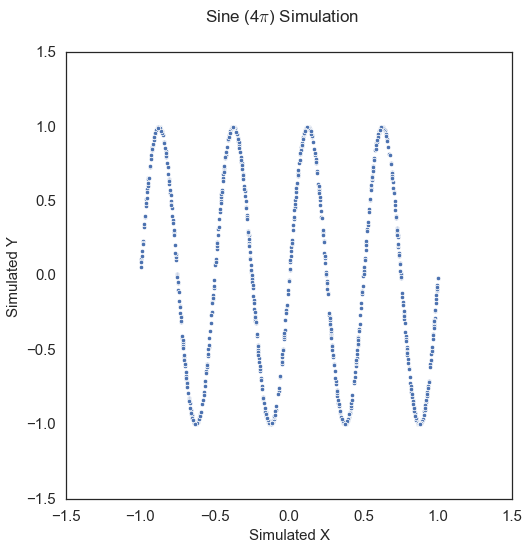
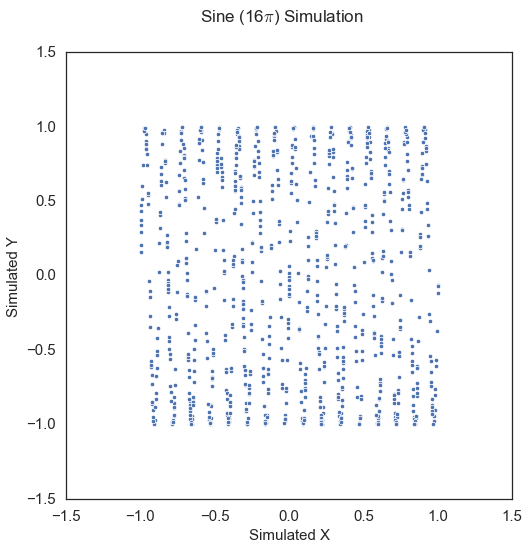
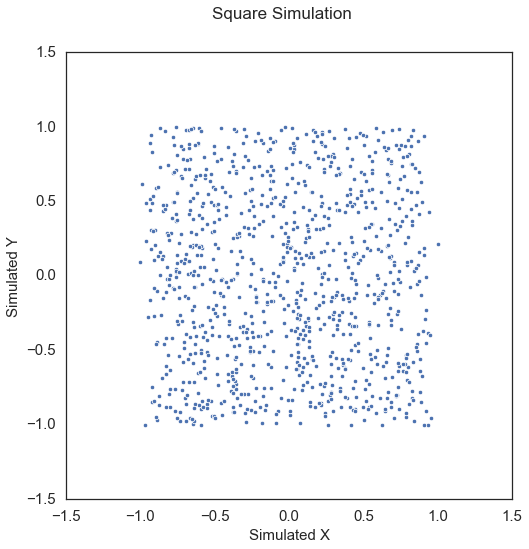
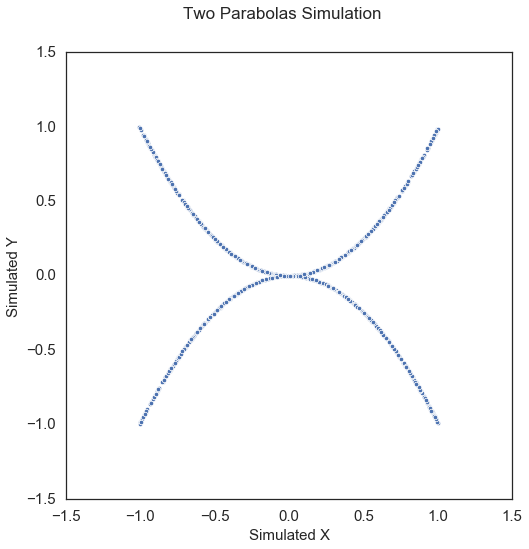
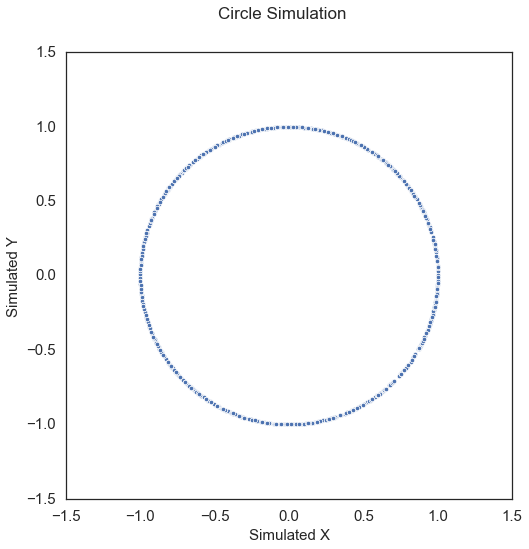
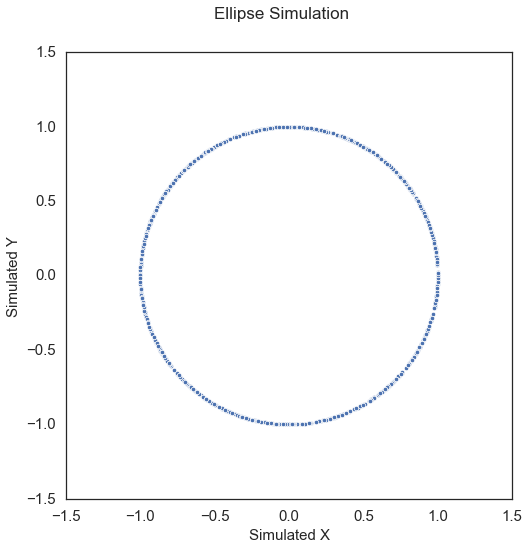
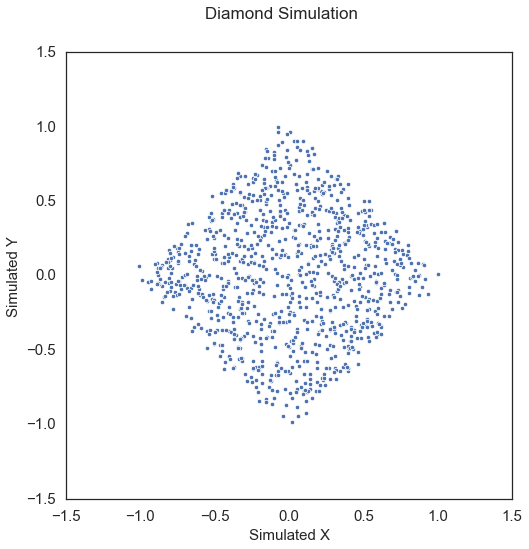
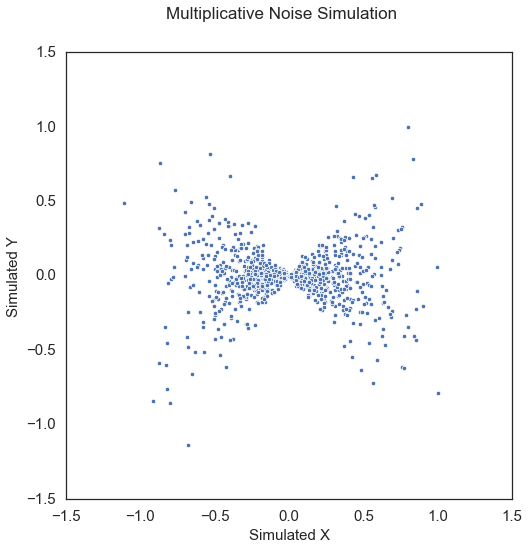
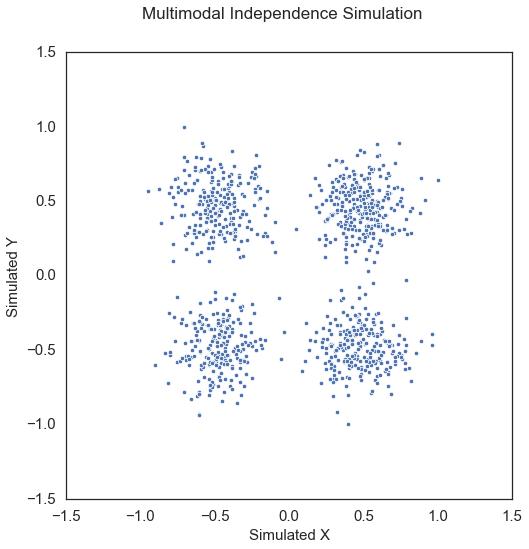